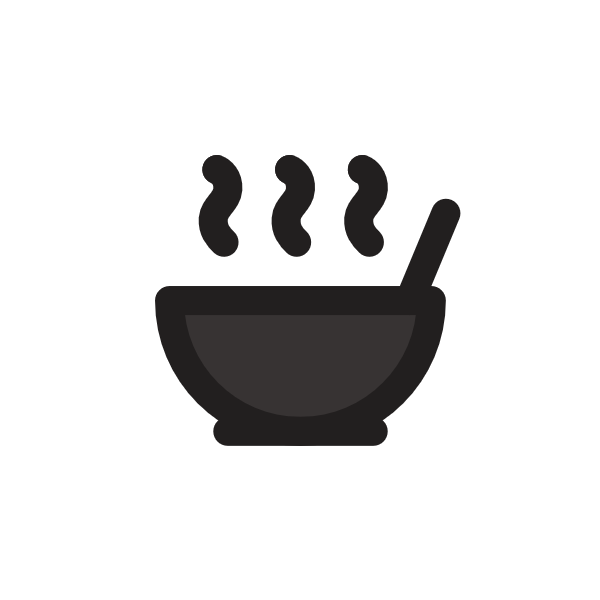
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Low-level programming language
Read the original article here.
Understanding Low-Level Programming: The Language of the Machine
In the pursuit of building a computer from scratch, understanding how software fundamentally interacts with hardware is paramount. This journey begins with exploring the world of low-level programming languages – the languages that live closest to the machine, offering direct control over its operations. Unlike high-level languages that abstract away hardware details, low-level languages expose the inner workings of the processor and memory, making them essential knowledge for anyone wanting to truly understand computing from the ground up.
What is a Low-Level Programming Language?
Let's start with a clear definition:
A low-level programming language is a programming language that provides little or no abstraction from a computer's instruction set architecture, memory, or underlying physical hardware. Commands or functions in the language are structurally similar to a processor's instructions.
Think of it this way: High-level languages (like Python, Java, C++, etc.) allow you to write code using concepts familiar to humans (variables, objects, complex data structures, loops that operate on lists), without needing to know exactly how the computer stores those variables, manages memory, or executes a loop step-by-step at the hardware level. Low-level languages, on the other hand, require you to think much closer to the hardware's perspective.
This proximity to the hardware has significant implications:
- Direct Control: Programmers have granular control over memory allocation, processor registers, and the exact sequence of operations the CPU performs.
- Performance: Programs written in low-level languages can be highly optimized for speed and efficient memory usage because the programmer dictates the low-level details.
- Non-Portability: Because they are so tied to the specific instruction set architecture (ISA) or even the operating system's low-level interfaces, programs written in low-level languages are typically not portable. Code written for an x86 processor won't run directly on an ARM processor, and vice-versa.
- Complexity: Writing and debugging low-level code is generally more difficult and time-consuming due to the lack of helpful abstractions. Every small operation must be explicitly coded.
Low-level languages are converted to machine code with minimal transformation, often directly (as with assembly) or through a simple assembly phase. They are fundamental building blocks in systems programming, operating system development, embedded systems, and performance-critical applications – areas you will inevitably touch upon when exploring how to build a computer from scratch.
The Foundation: Machine Code
At the absolute lowest level, software exists as machine code. This is the language that the Central Processing Unit (CPU) of a computer can directly understand and execute.
Machine code is the form in which code that can be directly executed is stored on a computer. It consists of machine language instructions, stored in memory, that perform operations such as moving values in and out of memory locations, arithmetic and Boolean logic, and testing values and, based on the test, either executing the next instruction in memory or executing an instruction at another location.
When you design or choose an Instruction Set Architecture (ISA) for your "from scratch" computer, you are defining the specific set of operations your CPU will understand. Machine code is the binary encoding of these operations.
For example, an instruction to add two numbers might be represented by a specific sequence of bits (e.g., 00000011
followed by bits indicating the source and destination). This sequence is read by the CPU's control unit, which then directs the arithmetic logic unit (ALU) and other components to perform the addition.
Machine code is stored in memory as binary data (sequences of 0s and 1s). While the computer works directly with binary, programmers often view machine code in hexadecimal representation for slightly better readability, as each hex digit conveniently represents four binary digits.
Why Programmers Don't Write Directly in Machine Code:
While machine code is what the computer executes, it is incredibly difficult for humans to read, write, and debug. Imagine trying to write an entire operating system or even a simple program using only sequences like 10110000 01100001
. It's tedious, error-prone, and nearly impossible to maintain.
However, understanding machine code is vital for deep debugging (analyzing memory dumps) or when working with very low-level bootstrapping processes where even an assembler isn't immediately available. Becoming adept at reading it, even if slowly, provides crucial insight into what the CPU is actually doing instruction by instruction.
Example: A snippet of x86-64 Machine Code (Hexadecimal Representation)
89 f8 85 ff 74 26 83 ff 02 76 1c 89 f9 ba 01 00 00 00 be 01 00 00 00 8d 04 16 83 f9 02 74 0d 89 d6 ff c9 89 c2 eb f0 b8 01 00 00 c3
This sequence represents a short function (specifically, calculating the nth Fibonacci number) encoded in the instruction set of an x86-64 processor. Each group of hex numbers (like 89 f8
) corresponds to a single machine instruction or part of one. Without a detailed lookup table or disassembler, it's almost impossible for a human to understand what this code does.
The First Step Up: Assembly Language
Since writing directly in machine code is impractical, the next level of abstraction is assembly language. It is considered a second-generation programming language because it provides a symbolic representation for the machine's instructions.
Assembly language has little semantics or formal specification, being only a mapping of human-readable symbols, including symbolic addresses, to opcodes, addresses, numeric constants, strings and so on.
Assembly language replaces the raw binary or hexadecimal codes with mnemonics – short, readable abbreviations for each machine instruction. For example, instead of remembering the binary code 00000011
for addition (in some hypothetical ISA), you might use the mnemonic ADD
.
Typically, there is a one-to-one correspondence between a line of assembly code (representing a single instruction) and a machine code instruction.
The Role of the Assembler:
A program called an assembler translates assembly language code into machine code.
An assembler is a utility program that processes assembly language source code into executable machine code.
The assembler reads the assembly mnemonics, symbolic addresses, and other symbols and converts them into the binary format specific to the target Instruction Set Architecture (ISA). The output of an assembler is often an object file, which contains the machine code along with information needed by a linker.
Advantages of Assembly Language:
- Readability: Much easier to read and write than raw machine code.
- Control: Still provides direct, granular control over hardware resources like registers and memory.
- Access: Allows access to specific processor instructions that might not be directly exposed by higher-level languages.
Disadvantages of Assembly Language:
- Architecture Dependent: Assembly language is specific to a particular ISA. Code written for an x86 processor will not work on an ARM processor.
- Tedious: Requires writing many lines of code to perform relatively simple tasks compared to high-level languages.
Context for "Building From Scratch":
When you build your own computer, the very first software you run (often called a bootloader or firmware) is frequently written in assembly language. You need assembly to:
- Initialize the CPU and other hardware components.
- Set up memory.
- Load the next stage of software (like an operating system kernel) into memory.
You also need an assembler that runs on another computer to translate your assembly source code into the machine code that your new hardware understands. Creating this initial toolchain is a crucial step in the "from scratch" process.
Example: The Same Fibonacci Function in x86-64 Assembly Language (Intel Syntax)
; Assuming function receives n in rdi
; Returns Fibonacci(n) in rax
fib:
; Base cases: if n <= 1, return n
cmp rdi, 1 ; Compare n (in rdi) with 1
jle .base_case ; If n <= 1, jump to .base_case
; Iterative calculation (for n > 1)
mov rax, 0 ; Initialize f(n-2) to 0 (fib(0))
mov rcx, 1 ; Initialize f(n-1) to 1 (fib(1))
mov r8, 2 ; Counter i = 2
.loop:
cmp r8, rdi ; Compare counter i with n
jg .done ; If i > n, jump to .done (loop finished)
; Calculate next Fibonacci number f(i) = f(i-1) + f(i-2)
mov r9, rcx ; Store f(i-1) in r9
add r9, rax ; Calculate f(i) = f(i-1) + f(i-2) (r9 = rcx + rax)
; Update f(n-2) and f(n-1) for the next iteration
mov rax, rcx ; New f(n-2) becomes old f(n-1)
mov rcx, r9 ; New f(n-1) becomes f(i)
inc r8 ; Increment counter i
jmp .loop ; Jump back to the start of the loop
.base_case:
mov rax, rdi ; If n <= 1, return n (n is already in rdi)
ret ; Return from function
.done:
mov rax, rcx ; The result fib(n) is in rcx (the last f(n-1))
ret ; Return from function
This example is much more readable than the raw hex. We see instructions like mov
(move data), cmp
(compare), jle
(jump if less than or equal), add
(add), inc
(increment), jmp
(jump), and ret
(return). We also see direct references to processor registers like rdi
, rax
, rcx
, r8
, r9
, which are small storage locations directly on the CPU used for calculations and data manipulation.
Notice how this code explicitly manages which register holds which value (f(n-2)
, f(n-1)
, counter, etc.). It also uses jump instructions (jle
, jmp
) to control the program flow, equivalent to loops and if-statements in higher-level languages.
Calling Conventions and ABIs:
The assembly example mentions the System V application binary interface for x86-64 (ABI). This highlights an important concept: while assembly language provides the instructions, the rules for how functions pass arguments, receive return values, and use system resources are often defined by a separate standard called an ABI.
An Application Binary Interface (ABI) is a set of rules and agreements about how programs interact at the binary level. This includes details like how function parameters are passed (e.g., in specific registers or on the stack), how return values are delivered, how system calls are made, how memory is laid out for data structures, and more.
Assembly language itself doesn't enforce ABIs. You could theoretically invent your own way to pass parameters. However, to interact with code compiled by standard compilers, or to write operating system components that other programs will use, you must adhere to the chosen ABI for your system. When building from scratch, you might initially define a simple custom ABI for your minimal system.
Bridging the Gap: C as a "Low-Level" High-Level Language
While assembly language gives ultimate control, it's impractical for writing large, complex software like operating systems or major applications. This is where languages like C come in. C is often described as a middle-level language or, as the source article points out, a low-level language when compared to most subsequently developed languages, yet high-level compared to assembly.
C provides crucial abstractions that assembly lacks:
- Variables: You can declare variables (
int n;
,long long f_nminus2;
) without specifying exactly which register or memory address they map to. The compiler makes this decision. - Data Types: C understands data types (integers, characters, pointers, structs), allowing the compiler to manage memory appropriately and perform type checking.
- Functions: C has a formal concept of functions with defined parameters and return types. The compiler, following the ABI, handles the low-level details of passing arguments and returning values.
- Control Structures: C provides structured control flow (
if
,else
,for
,while
) which are translated into low-level jumps and conditional branches by the compiler.
Why C is Still Considered Low-Level:
Despite these abstractions, C is considered low-level compared to many languages because:
- Direct Memory Access: C provides pointers, which are variables that store memory addresses. This allows direct manipulation of specific memory locations, similar to how assembly works with addresses, albeit with some type safety. This feature is crucial for low-level tasks like hardware interaction and memory management.
- Manual Memory Management: C requires the programmer to manually allocate and deallocate memory using functions like
malloc
andfree
. There's no built-in garbage collection like in Java or Python. This gives the programmer explicit control but also introduces the risk of memory leaks or errors. - Close to Hardware Concepts: C's structure maps relatively closely to typical processor architectures. Concepts like arrays mapping directly to contiguous memory blocks are evident.
Context for "Building From Scratch":
C is incredibly important in the world of building systems from scratch because:
- Operating System Development: Most modern operating systems (Linux, Windows kernels, macOS kernel) are written primarily in C.
- Compilers and Tools: The compilers and assemblers needed for your new architecture are often written in C.
- Hardware Abstraction: C allows you to write code that interacts with hardware (via pointers to specific memory-mapped registers) while still using higher-level language features for overall program structure.
- Portability: While C code needs a specific compiler for each architecture, the source code is often portable. You can write a C program once and compile it for your new architecture, provided you have a C compiler targeting that architecture. This is a massive step up from assembly.
Example: The Same Fibonacci Function in C
long long fib(int n) {
if (n <= 1) {
return n;
} else {
long long f_nminus2 = 0; // Represents fib(0)
long long f_nminus1 = 1; // Represents fib(1)
long long f_n;
int i = 2;
while (i <= n) {
f_n = f_nminus1 + f_nminus2;
f_nminus2 = f_nminus1;
f_nminus1 = f_n;
i++;
}
return f_nminus1; // The last f_nminus1 is fib(n)
}
}
Comparing this C code to the assembly and machine code examples, the increase in abstraction is clear. Variables (n
, f_nminus2
, etc.) are named symbolically, arithmetic operations (+
) are expressed directly, and control flow (if
, while
) is represented by structured keywords. The programmer doesn't specify which register holds f_nminus2
or how the loop jump is implemented; the C compiler handles those low-level details.
Low-Level Programming in High-Level Languages (Inline Assembly)
Even when working primarily in a higher-level language like C, there are sometimes needs to drop down to the machine level for specific tasks. Modern languages sometimes offer features to accommodate this.
Historically, languages designed for systems programming in the late 1960s and 1970s (like PL/S, BLISS, BCPL, C) started including features that allowed some degree of low-level access within a higher-level syntax.
One common technique for this is inline assembly.
Inline assembly is a feature provided by some compilers and languages that allows assembly language code to be embedded directly within source code written in a higher-level language.
This lets programmers insert architecture-specific assembly instructions at critical points within their C code, for instance, to optimize a small, performance-sensitive loop, access specific hardware instructions not exposed by C, or implement parts of the operating system kernel that must run at a privileged level.
Context for "Building From Scratch":
When writing the core components for your custom hardware (e.g., interrupt handlers, context switching code in an OS kernel, very specific I/O operations), you might find yourself needing to use inline assembly within your C code. This allows you to leverage the structure and convenience of C for most of the code while retaining the ability to perform essential, low-level hardware manipulations where necessary.
Example: C code snippet using GCC Inline Assembly (x86)
/* Simple copy and add using inline assembly */
/* From GCC documentation examples */
int src = 1;
int dst;
int val = 5;
__asm__ __volatile__ (
"mov %1, %0\n\t" // Move the value from src (%1) into dst (%0)
"add %2, %0" // Add the value from val (%2) to dst (%0)
: "=r" (dst) // Output: dst is the output operand (%0), "r" means use any general-purpose register
: "r" (src), // Input: src is the first input operand (%1), "r" means use any general-purpose register
"r" (val) // Input: val is the second input operand (%2), "r" means use any general-purpose register
: // Clobbered registers (none explicitly clobbered here)
);
// After this block, dst will hold (src + val), i.e., 1 + 5 = 6
This example shows how the __asm__ __volatile__ (...)
syntax in GCC (a common C compiler) allows embedding assembly instructions (mov
, add
). The syntax with %0
, %1
, %2
, and the : "=r" (dst)
sections is specific to how GCC handles inline assembly, allowing the programmer to specify which C variables map to which assembly operands (typically registers, indicated by "r"
). This demonstrates a direct interaction between the C language's variables and the processor's low-level operations.
Why Understanding Low-Level Programming Matters for Building From Scratch
Mastering low-level programming is not just an academic exercise when your goal is to build a computer from the ground up. It's a fundamental requirement because:
- You Define the Hardware: You are designing or deeply interacting with the Instruction Set Architecture. You need to understand how instructions are encoded (machine code) and how they are represented symbolically (assembly) because you will implement these in hardware and then write the first software that uses them.
- Bootstrapping: The initial process of getting your computer running – loading programs, setting up memory – requires writing code without the luxury of an existing operating system or runtime environment. This code is necessarily low-level (assembly or minimal C).
- Hardware Interaction: To make your hardware do anything useful, you need to write code that directly controls input/output devices, memory controllers, and other peripherals. This often involves writing to specific memory addresses or ports, a task handled by low-level code.
- Building the Software Stack: Even if you plan to eventually run high-level languages, you first need to build the layers beneath: the bootloader, potentially a simple operating system kernel, device drivers, and the tools (assembler, compiler) that translate higher-level code into your hardware's machine code. All these layers rely heavily on low-level programming.
- Deep Debugging: When hardware or fundamental software issues arise, you'll need to be able to analyze the state of the machine at the most basic level – inspecting memory contents and understanding machine code execution flow.
In essence, low-level programming languages are the bridge between the hardware you build and the software you want to run on it. They provide the necessary control and insight to bring a computer to life from its most fundamental components.